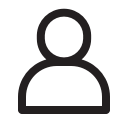
KNN(K Nearest Neighbors)
This is my final version of K Nearest Neighbors. I still may need to add more points.
This is my final version of K Nearest Neighbors. I still may need to add more points.
The posts I have seen have been great and many of them are focused on algorithms - correlation between stocks, decision trees, k nearest neighbors. I wanted to start a thread regarding where your work can be applied to. When I think of the k nearest neighbors algorithm, I think of pattern recognition. Using data to predict how often you make large purchases, how weather conditions will affect the extent of your allergies, etc. As you progress to more complex algorithms like SVM and K Means Clustering, let us know what cases you see these algorithms being used.
This is my KNN code
Test message from nexclap - please ignore
2023-02-13
Test message from nexclap - please ignore
2023-02-11
Test message from nexclap - please ignore
2023-02-11