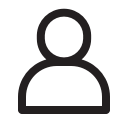
Rock Paper Scissors
This is a game of rock paper scissors with the CPU. The user is prompted to choose rock, paper, or scissor and then the CPU randomly selects one of the three. Afterwards the winner is outputted to the console. The main part of the code was the nested "if" statements to check for all the possible outcomes that could result between the user and the CPU.